toString() : 在处理数字的时候,接受一个进制参数,默认十进制
var age = 11;
var ageAsString = age.toString(); //the string "11"
var found = true;
var foundAsString = found.toString(); //the string "true"
// 对数字的处理,可以特殊一点
var num = 10;
alert(num.toString()); //"10"
alert(num.toString(2)); //"1010"
alert(num.toString(8)); //"12"
alert(num.toString(10)); //"10"
alert(num.toString(16)); //"a"
fromCharCode(); // 把asiic 对应的数字转换为字符串
alert(String.fromCharCode(104, 101, 108, 108, 111)); //"hello"
indexOf() 与 lastIndexOf() 可以参考数组的用法
slice()、substring()、substr() 截取字符串
var stringValue = "hello world";
alert(stringValue.slice(3)); //"lo world"
alert(stringValue.substring(3)); //"lo world"
alert(stringValue.substr(3)); //"lo world"
alert(stringValue.slice(3, 7)); //"lo w"
alert(stringValue.substring(3,7)); //"lo w"
alert(stringValue.substr(3, 7)); //"lo worl"
alert(stringValue.slice(-3)); //"rld"
alert(stringValue.substring(-3)); //"hello world"
alert(stringValue.substr(-3)); //"rld"
alert(stringValue.slice(3, -4)); //"lo w"
alert(stringValue.substring(3, -4)); //"hel"
alert(stringValue.substr(3, -4)); //"" (empty string)
String 强制转换
var value1 = 10;
var value2 = true;
var value3 = null;
var value4;
alert(String(value1)); //"10"
alert(String(value2)); //"true"
alert(String(value3)); //"null"
alert(String(value4)); //"undefined"
转换大小写
var stringValue = "hello world";
alert(stringValue.toLocaleUpperCase()); //"HELLO WORLD"
alert(stringValue.toUpperCase()); //"HELLO WORLD"
alert(stringValue.toLocaleLowerCase()); //"hello world"
alert(stringValue.toLowerCase()); //"hello world"
声明方法的不同,导致类型不同
var stringObject = new String("hello world");
var stringValue = "hello world";
console.log(typeof stringObject); //"object"
console.log(typeof stringValue); //"string"
console.log(stringObject instanceof String); //true
console.log(stringValue instanceof String); //false
字符串的正则操作:match()、search()、replace()、split()
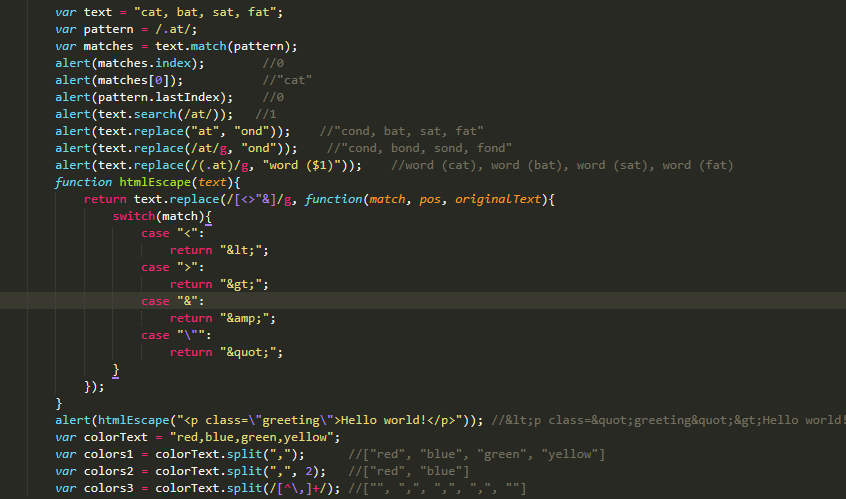